Java Tutorial
IntroductionEnvironment SetupIDEBuild ManagementLanguage SpecificationBasic ProgramVariablesData TypesPackagesModifiersConditionalsLoopsObject OrientedClassesSuperInterfacesEnumStatic importInheritanceAbstractionEncapsulationPolymorphismBoxing & UnboxingConversion Formatting numbers Arrays Command line arguments in java Variable Number of arguments in Java Exception handling in Java String handling in Java StringBuffer and StringBuilder in Java Mathematical Operations in Java Date and Time in Java Regular expressions in Java Input output programming in Java File Handling Nested Classes Collections Generics Serialization Socket programming Multi-Threading Annotations Lambda Expressions Reflections in Java Singleton class in Java Runtime Class in JavaHow to load resource in JavaHow to load properties file in JavaAdvanced
Log4j – Logging framework in JavaInterview Questions in JavaThere are 2 types of sockets in Java.
Communicating over tcp/udp is possible through ServerSocket class. Server socket will do below tasks.
- ServerSocket – TCP
- DatagramSocket – UDP
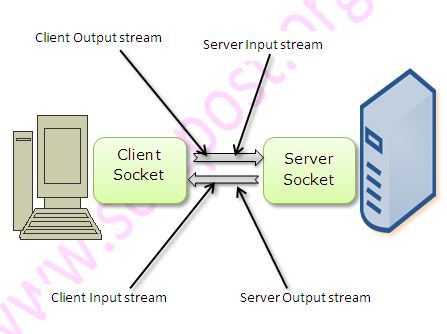
- Create a Server Socket using ServerSocket Class
- Then call accept() method to wait for connections
- Then read and write to sockets i/p & o/p streams
- Connect to server using below syntax
- Socket client = new Socket(serverName, port);
- Then read and write to socket using i/p & o/p streams
package sockets;
/**
* Created by ssalunke on 12/04/2016.
*/
import java.net.*;
import java.io.*;
public class Server extends Thread {
public static void main(String[] args) throws Exception {
System.out.println("Server Listening on 8888");
ServerSocket serverSocket = new ServerSocket(8888);
//server timeout 60 minutes
serverSocket.setSoTimeout(1000 * 60 * 60);
//Below method waits until client socket tries to connect
Socket server = serverSocket.accept();
//Read from client using input stream
DataInputStream in = new DataInputStream(server.getInputStream());
System.out.println(in.readUTF());
//Write to client using output stream
DataOutputStream out = new DataOutputStream(server.getOutputStream());
out.writeUTF("Bye sent by Server");
//close the connection
server.close();
}
}
Here is the client code
package sockets;
import java.net.*;
import java.io.*;
public class Client {
public static void main(String[] args) throws Exception{
//try to connect to server - localhost @ port 8888
Socket client = new Socket("localhost",8888);
//if server is not listening - You will get Exception
// java.net.ConnectException: Connection refused: connect
//write to server using output stream
DataOutputStream out = new DataOutputStream(client.getOutputStream());
out.writeUTF("Hello server - How are you sent by Client");
//read from the server
DataInputStream in = new DataInputStream(client.getInputStream());
System.out.println("Data received from the server is -> " + in.readUTF());
//close the connection
client.close();
}
}
Here is the output of above example.
Please run the server code first then run client code.
Here is the server output.
Server Listening on 8888
Hello server – How are you sent by Client
Here is the client output.
Data received from the server is -> Bye sent by Server
Web development and Automation testing
solutions delivered!!