Java Tutorial
IntroductionEnvironment SetupIDEBuild ManagementLanguage SpecificationBasic ProgramVariablesData TypesPackagesModifiersConditionalsLoopsObject OrientedClassesSuperInterfacesEnumStatic importInheritanceAbstractionEncapsulationPolymorphismBoxing & UnboxingConversion Formatting numbers Arrays Command line arguments in java Variable Number of arguments in Java Exception handling in Java String handling in Java StringBuffer and StringBuilder in Java Mathematical Operations in Java Date and Time in Java Regular expressions in Java Input output programming in Java File Handling Nested Classes Collections Generics Serialization Socket programming Multi-Threading Annotations Lambda Expressions Reflections in Java Singleton class in Java Runtime Class in JavaHow to load resource in JavaHow to load properties file in JavaAdvanced
Log4j – Logging framework in JavaInterview Questions in JavaIn this topic, we will learn what is Serialization, how we can achieve it and what are the advantages of it. Serialization is nothing but storing the object on disk, memory or database in the form of bytes. Main advantage of Serialization is that we can restore the object in future. The reverse process of getting object from disk or file is called as Deserialization.
Remember that transient members are not saved during Serialization process. Below example illustrates how we can perform Serialization and Deserialization.
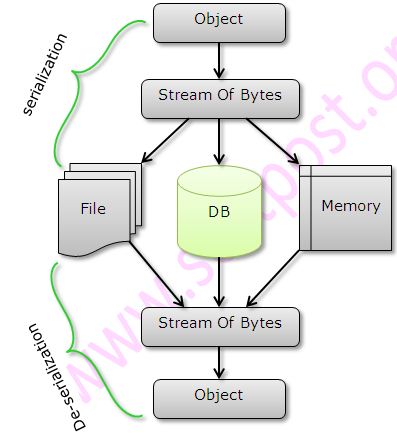
package serialization;
/**
* Created by ssalunke on 12/04/2016.
*/
import java.io.*;
public class Serialization
{
public static void main(String [] args)
{
Student student = new Student();
student.name = "Sagar Salunke";
student.rollno = 10;
student.tempNo = 111;
try
{
FileOutputStream fileOut = new FileOutputStream("student.obj");
ObjectOutputStream out = new ObjectOutputStream(fileOut);
out.writeObject(student);
out.close();
fileOut.close();
System.out.println("Student object will be stored in student.obj file");
}catch(IOException i)
{
i.printStackTrace();
}
}
}
class DeserializeStudent
{
public static void main(String [] args)
{
Student student = null;
try
{
FileInputStream fileIn = new FileInputStream("student.obj");
ObjectInputStream in = new ObjectInputStream(fileIn);
student = (Student) in.readObject();
in.close();
fileIn.close();
}catch(IOException i)
{
i.printStackTrace();
return;
}catch(ClassNotFoundException c)
{
System.out.println("Student class not found");
c.printStackTrace();
return;
}
System.out.println("Deserialized Student...");
System.out.println("Name -> " + student.name);
System.out.println("Roll no -> " + student.rollno);
System.out.println("Temp no -> " + student.tempNo);
}
}
class Student implements java.io.Serializable
{
//transient members will not be saved
public int rollno;
public String name;
public transient int tempNo;
}
Here is the output of above example.
Deserialized Student…
Name -> Sagar Salunke
Roll no -> 10
Temp no -> 0
Web development and Automation testing
solutions delivered!!