Java Tutorial
IntroductionEnvironment SetupIDEBuild ManagementLanguage SpecificationBasic ProgramVariablesData TypesPackagesModifiersConditionalsLoopsObject OrientedClassesSuperInterfacesEnumStatic importInheritanceAbstractionEncapsulationPolymorphismBoxing & UnboxingConversion Formatting numbers Arrays Command line arguments in java Variable Number of arguments in Java Exception handling in Java String handling in Java StringBuffer and StringBuilder in Java Mathematical Operations in Java Date and Time in Java Regular expressions in Java Input output programming in Java File Handling Nested Classes Collections Generics Serialization Socket programming Multi-Threading Annotations Lambda Expressions Reflections in Java Singleton class in Java Runtime Class in JavaHow to load resource in JavaHow to load properties file in JavaAdvanced
Log4j – Logging framework in JavaInterview Questions in JavaNow let us try to understand interfaces in Java. Interfaces provide specification or standard. They tell what must be done. But they do not specify how it is to be done or implemented. Another class has to provide the implementation. Interfaces kind of create a standard set of methods as all classes implementing that interfaces must implement method with the same name. Interfaces are like Classes in terms of syntax. Major difference between interfaces and classes are –
Example – In below example, we have a Movable interface with one method declaration move() and a variable MAX_SPEED. Class Bike implements that interface by providing definition of the method move().
- Interfaces contain only declaration of method. Concrete classes must have definitions of the methods. Abstract classes may contain undefined methods.
- An interface can extend another interface (not a class) and can implement multiple interfaces. A class can implement multiple interfaces but can just extend one class.
- All members of Interface are public by default.
- Interfaces variables are final and static by default.
- All methods of the Interface are abstract by default.
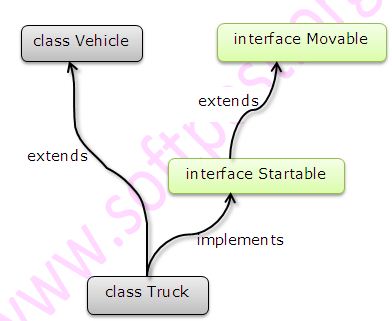
package oops;
/**
* Created by Sagar on 16-04-2016.
*/
public class interface_implementation {
public static void main(String [] args){
Bike kawasaki = new Bike();
kawasaki.move();
}
}
class Bike implements Movable{
@Override
public void move() {
System.out.println("Move implementation");
System.out.println("Max speed can be -> " + Movable.MAX_SPEED);
}
}
interface Movable {
int MAX_SPEED = 100;
void move();
}
Here is the output of Above Java example.
Move implementation
Max speed can be -> 100
Web development and Automation testing
solutions delivered!!