Java Tutorial
IntroductionEnvironment SetupIDEBuild ManagementLanguage SpecificationBasic ProgramVariablesData TypesPackagesModifiersConditionalsLoopsObject OrientedClassesSuperInterfacesEnumStatic importInheritanceAbstractionEncapsulationPolymorphismBoxing & UnboxingConversion Formatting numbers Arrays Command line arguments in java Variable Number of arguments in Java Exception handling in Java String handling in Java StringBuffer and StringBuilder in Java Mathematical Operations in Java Date and Time in Java Regular expressions in Java Input output programming in Java File Handling Nested Classes Collections Generics Serialization Socket programming Multi-Threading Annotations Lambda Expressions Reflections in Java Singleton class in Java Runtime Class in JavaHow to load resource in JavaHow to load properties file in JavaAdvanced
Log4j – Logging framework in JavaInterview Questions in JavaThere are 2 main types of nested class.
Non-static (Inner class) classes can be divided into 3 types as mentioned below.
- Static nested class
- Non-Static nested class (Also called as Inner class)
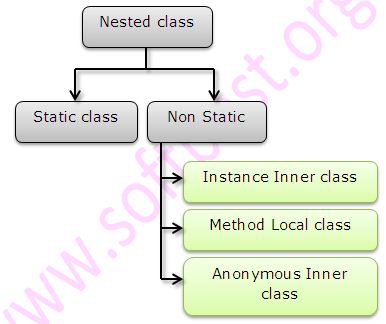
- Normal
- Method local
- Anonymous
package corejava.nestedclasses;
//Class A is an outer class
public class A {
private int number;
public String name;
static private String city;
//class B is an inner class (Non-Static)
class B {
public void printB(){
System.out.println("This is non-static nested class " +
"so it can access private members of outer class" + name);
}
}
public void hi() {
//Class C is a method local inner class
class C {
}
C c1 = new C();
}
//Static class
static class D{
public void print(){
System.out.println("This is static inner class");
System.out.println("I can not access non static " +
"members of outer but can access static memebrs" +
"like city in this case");
//We can not access class C from here
// as it is local to method hi() of outer class only
//C c1 = new C();
}
}
public static void main(String[] args) {
D d1 = new D();
d1.print();
}
}
Web development and Automation testing
solutions delivered!!