Java Tutorial
IntroductionEnvironment SetupIDEBuild ManagementLanguage SpecificationBasic ProgramVariablesData TypesPackagesModifiersConditionalsLoopsObject OrientedClassesSuperInterfacesEnumStatic importInheritanceAbstractionEncapsulationPolymorphismBoxing & UnboxingConversion Formatting numbers Arrays Command line arguments in java Variable Number of arguments in Java Exception handling in Java String handling in Java StringBuffer and StringBuilder in Java Mathematical Operations in Java Date and Time in Java Regular expressions in Java Input output programming in Java File Handling Nested Classes Collections Generics Serialization Socket programming Multi-Threading Annotations Lambda Expressions Reflections in Java Singleton class in Java Runtime Class in JavaHow to load resource in JavaHow to load properties file in JavaAdvanced
Log4j – Logging framework in JavaInterview Questions in JavaException is an unexpected event that might occur at the time of executing the program. For example – if you are trying to open a file with name xyz.txt and that file does not exist on disk. At that time, you will get Exception viz. FileNotFoundException So if you think some part of code is risky and might throw Exception, you need to handle it so that program will continue to run in graceful manner. In Java, we can use try…..catch construct to handle the Exceptions.
There are 2 types of Exceptions in Java.
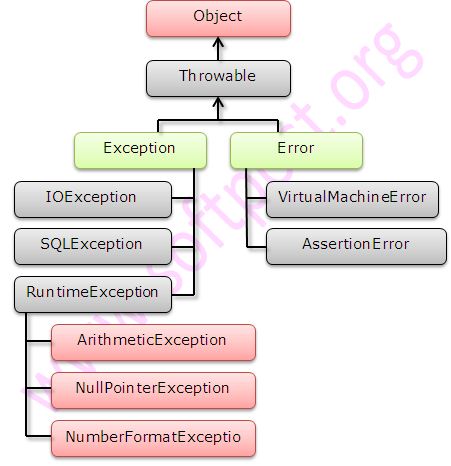
- Checked Exception
- Unchecked Exception
throw and throws keyword in Java
throw keyword is used to throw custom exception. throws keyword is used when you do not want to handle the exception in current method. In this case, the caller of the method will have to handle the exception. Below example illustrates how we can use try ….catch to handle exception.
package exceptions;
import org.testng.reporters.Files;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.IOException;
/**
* Created by Sagar on 16-04-2016.
*/
public class TestExceptions {
public static void main(String [] args) {
try {
Files.writeFile("shs",new File("c:\xx"));
} catch (FileNotFoundException ex) {
System.out.println("File not found Exception occured");
ex.printStackTrace();
}catch (IOException ex){
ex.printStackTrace();
}finally {
System.out.println("This finally block of code will be executed"
+ " No matter if Exception is thrown or not");
}
System.out.println("Program continuing even after Exception comes");
//Unchecked Exception - ArithmeticException
//If you comment below line, program will exit with code 0
System.out.println("this line throws " + 11/0);
}
}
Here is the output of above example.
This finally block of code will be executed No matter if Exception is thrown or not
Program continuing even after Exception comes
Process finished with exit code 1
File not found Exception occured
java.io.FileNotFoundException: c: (Access is denied)
Exception in thread “main” java.lang.ArithmeticException: / by zero
This finally block of code will be executed No matter
if Exception is thrown or not
Program continuing even after Exception comes
Web development and Automation testing
solutions delivered!!