Cucumber + Java
Introduction to Cucumber Installation of CucumberAdding Cucumber dependency to Java project Writing first cucumber test Executing Cucumber tests using Cucumber class Cucumber options Tagging the scenarios Using name option Passing parameters to steps Key – Value pair Datatable Multiple column datatable in Cucumber Scenario Background Scenario outline Running multiple feature files in Cucumber Sharing selenium Webdriver instance using PicoContainer Embedding the screenshot Writing to Cucumber HTML reports Cucumber dependency for using Lambda expressions in Java 8 Cucumber test using Lambda expressionsWriting first cucumber test
Just follow below steps to write first cucumber test in IntelliJ IDEA.- Create a maven project.
- Add feature file containing scenarios in Test Resources directory.
- Add the maven dependency for Cucumber.
- Write step definitions and execute the tests from feature file.
@selenium
Feature: My feature
Scenario: Verify title
Given I am on the www.softpost.org home page
Then I verify that title contains tutorials
Note that we have not added the step definitions for above steps in feature file. When you execute the feature file, you will see below errors. You can implement missing steps with the snippets below:
@Given(“^I am on the www\.softpost\.org home page$”)
public void i_am_on_the_www_softpost_org_home_page() throws Throwable {
// Write code here that turns the phrase above into concrete actions
throw new PendingException();
}
@Then(“^I verify that title contains tutorials$”)
public void i_verify_that_title_contains_tutorials() throws Throwable {
// Write code here that turns the phrase above into concrete actions
throw new PendingException();
}
Undefined step: Given I am on the www.softpost.org home page
Undefined step: Then I verify that title contains tutorials
Writing the step definitions
You can copy above method snippets and write your own code inside it. Alternatively, if you have cucumber for Java and Gherkin plugin installed in IntelliJ IDEA, then you can create the step definitions by pressing alt + enter on the steps in Feature file as shown in below image.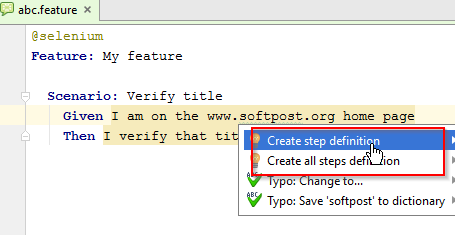
package org.softpost;
import cucumber.api.java.After;
import cucumber.api.java.Before;
import cucumber.api.java.en.Given;
import cucumber.api.java.en.Then;
import junit.framework.Assert;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
/**
* Created by Sagar on 12-07-2016.
*/
@SuppressWarnings("ALL")
public class seleniumsteps {
WebDriver driver;
@Before
public void launchBrowser(){
driver = new FirefoxDriver();
}
@Given("^I am on the www\.softpost\.org home page$")
public void i_am_on_the_www_softpost_org_home_page() throws Throwable {
driver.get("https://www.softpost.org");
}
@Then("^I verify that title contains tutorials$")
public void i_verify_that_title_contains_tutorials() throws Throwable {
Assert.assertTrue(driver.getTitle().toLowerCase().contains("tutorials"));
}
@After
public void killBrowser(){
driver.close();
driver.quit();
}
}
After writing the step definitions, you can create the run configuration for feature file as shown in below image. Note that Glue property is very important. You should specify the package name that contains step definitions in the Glue.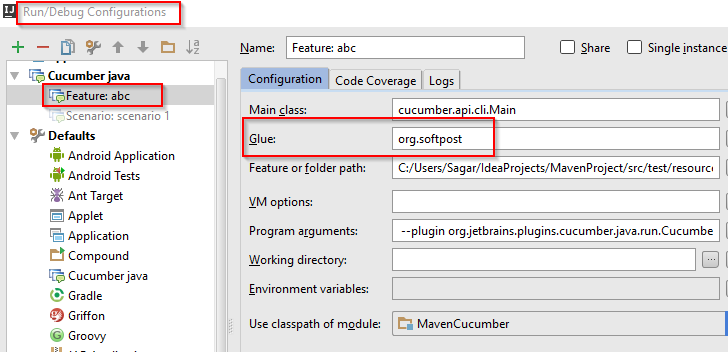
Web development and Automation testing
solutions delivered!!